Language Features I Wish JavaScript Had
The wonders of guard and pattern matching
2 minute read
( )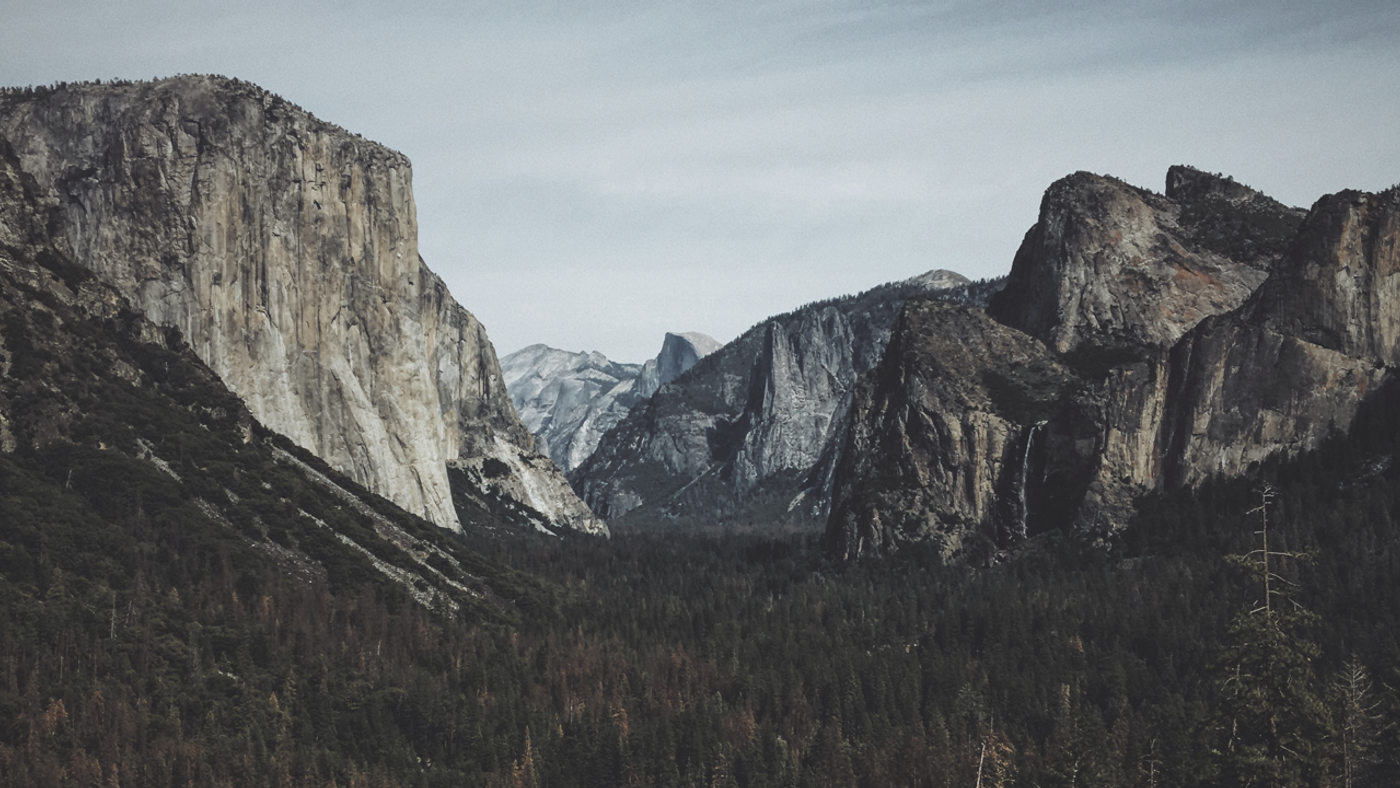
This post is also available in different formats so you can read on the go or share it around!
JavaScript is much maligned by developers who use “real languages”, but, for all the quirks and shortcomings, I still love JavaScript. That isn’t to say that JavaScript is perfect but it gets the job done when you need it and the community around JavaScript is flourishing. With that said, spending some time in Elixir, the grass is always greener on the other side and there are some language features that I wish JavaScript had.
Elixir is a dynamic functional language with a couple of things I really enjoy using, pattern matching and guards are the coolest things I’ve seen.
Pattern Matching
A staple of functional programming, pattern matching in the Elixir language is no different. It's something that I wish I could use in JavaScript as it makes certain tasks easier to understand and more concise. The easiest way to think about it is JavaScript's switch statement but infinitely more flexible.
What is pattern matching?
Pattern matching is one of the most confusing concepts for a newcomer to the language but it doesn’t take long to realize why it’s so powerful, you’ll be asking yourself how you’d ever lived without it.
Why is it useful?
Pattern matching is the most powerful feature I’ve come to enjoy when using Elixir. It makes code more understandable and infinitely more modular.
![Basic pattern matching on an array def color([red, green, blue]) do "rgb(#red, #green, #blue)" end](https://static-sethcorker.imgix.net/build/2018-04-30-language-features-i-wish-javascript-had/1*8aqLUS684RFn_BjFHmYLYw.png)
By applying pattern matching, we can extract the contents of the array directly and start using them. In the example above, ‘color/1’ can be called with two different lengths of array.
color([125, 223, 125]) # rgb(125, 223, 125)
color([125, 223, 125, 1]) # rgba(125, 223, 125, 1)
There are alternatives in JavaScript however they aren’t as concise or easy to understand at a glance. Pattern matching can be used throughout Elixir and often replaces conditionals which makes for understandable data flows. Pattern matching can be further enhanced with the use of Guards.
Guards
What are Guards?
Guards work in combination with pattern matching in Elixir to determine which function if any will match and respond to the caller.
How are Guards useful?
Guards are a great way to be more specific with pattern matching and produce much cleaner results than a switch statement of if/else chain in JavaScript.
defmodule PrettyMessage do
@moduledoc """
Documentation for PrettyMessage.
"""
def prettify(:error, number) when number > 3 do
"😱 Look at all those errors. You should be ashamed!"
end
def prettify(:error, number) when number == 1 do
"😦 Your have an error! Better get on that."
end
def prettify(:error, number) do
"😯 You've got #{number} errors, you might want to check those out."
end
def prettify(:ok) do
"👌 Everything looks okay top me."
end
end
A simple example showing guards and pattern matching
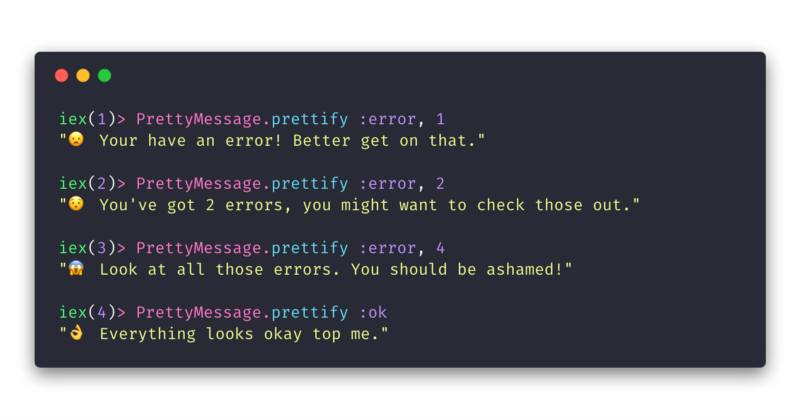
Another example
With a combination of pattern matching and simple guards, a module’s functions can be made easier to use from an outside perspective.
# Two functions that conceptually do the same thing
def takeFirstFromString(aString) do ... end
def takeFirstFromArray(anArray) do ... end
# Why not try pattern matching?
def takeFirst(col) do ... end
def takeFirst(col) when is_list(col) do ... end
# So when the from the user's perspective, it's one easier
takeFirst("Once")
takeFirst(["A", "B", "C"])
To learn more about how to use Guards in Elixir, Elixir’s documentation is a great source of knowledge with helpful examples and how best to use Guards to your advantage.
As JavaScript matures, it’s interesting to see influences from other languages slowly make their way into the common JavaScript vernacular. We already have some useful features in ES6 like destructing which is making code more readable and concise. I hope that we’ll get something as cool and useful as pattern matching or some other mainstays of functional programming languages.
Thanks for taking the time to let me delve into some cool Elixir language features. Let me know what other cool features Elixir has that you think would be cool to see in JavaScript.
Header photo by Jeff Finley on Unsplash
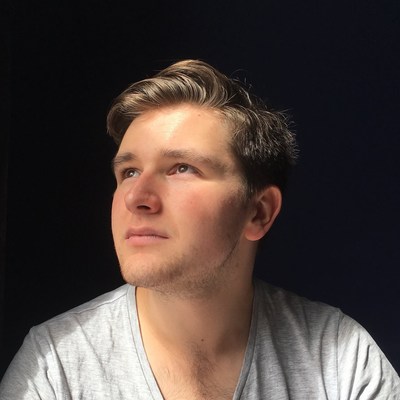
A Fullstack Software Engineer working with React and Django. My main focus is JavaScript specialising in frontend UI with React. I like to explore different frameworks and technologies in my spare time. Learning languages (programming and real life) is a blast.