A look at the React Context API
A useful addition to your React toolkit
3 minute read
( )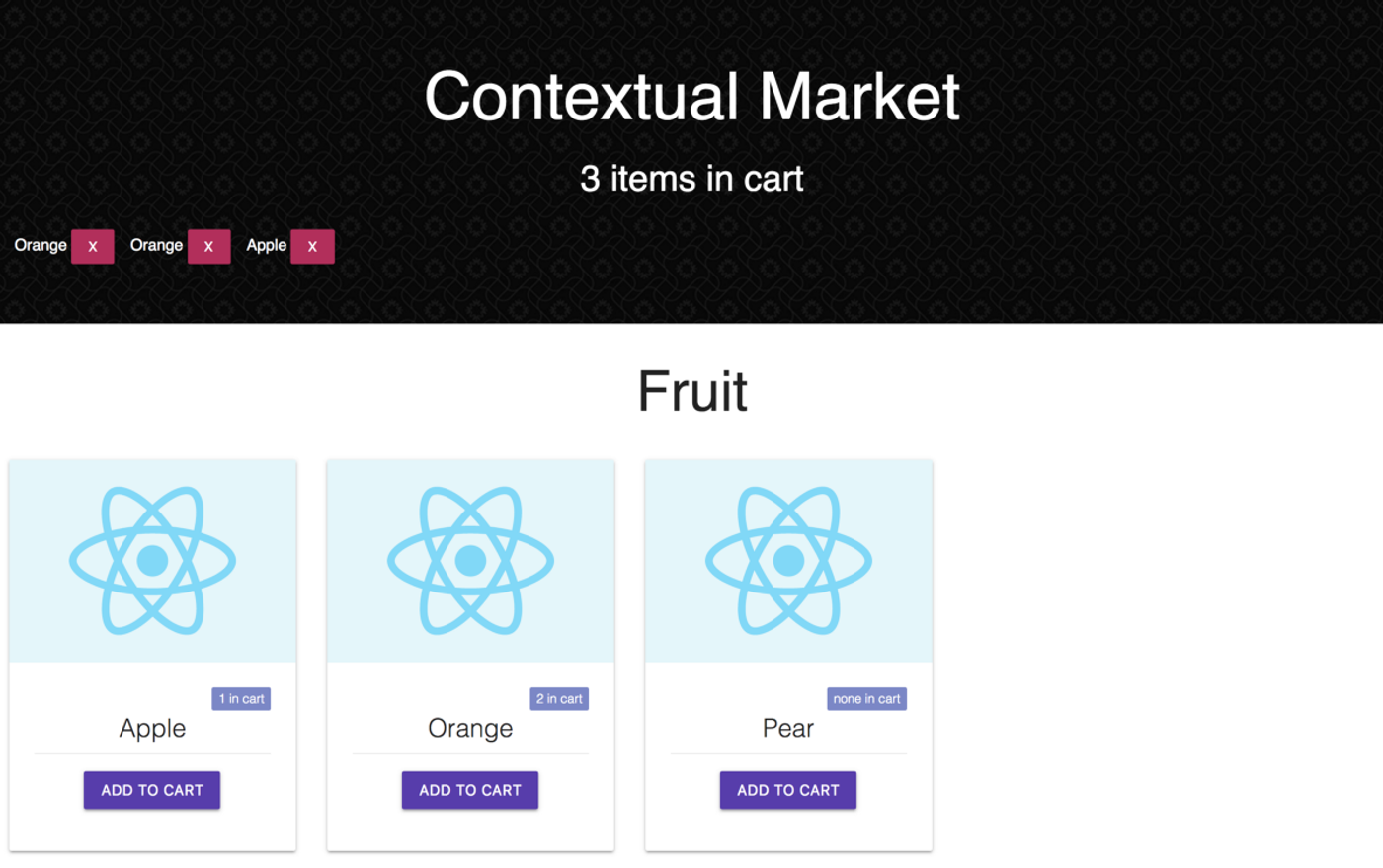
This post is also available in different formats so you can read on the go or share it around!
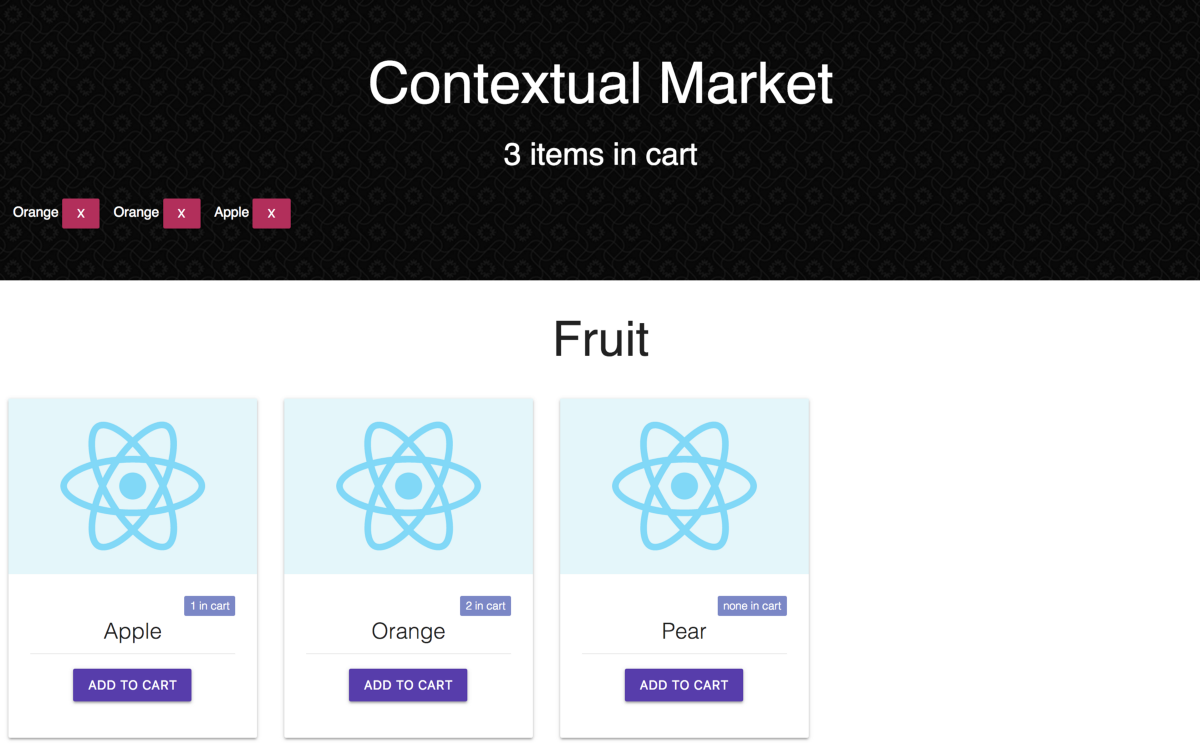
What is the Context API?
The API consists of two important components, the <Provider>
and the <Consumer>
. The Provider is tasked with “providing” a value which the Consumer can then access if it is a child in the tree.
Will the Context API replace Redux?
If you are using Redux just to avoid passing props down the tree, the React Context API could replace Redux. If you are just using Redux to circumvent prop-drilling, you might be missing out on the other features Redux has to offer. Redux is a different way of thinking about state management which encompasses a how data moves through your application, how it’s transformed and what the state of your application should be at any given moment.
The benefits of using Redux come in to play with the dev tools, the current state of an application can be derived from the actions which have been sent up until this point. The advantage of this is that bugs related to state can be identified easier, a QA could get into the exact state which fails and then send the action log to a developer to reproduce. That isn’t to say that alternatives to Redux which use this new API won’t crop up but I think it’s safe to say Redux is still an invaluable tool when used in and outside of the React framework.
How do I use it?
To demonstrate how this works, I made a basic sample which uses the new API, the full source is available on GitHub. The app will be a shopping cart where products can be added to a cart. A Provider can be placed near the root of the tree like this:
A sample using the new React Context API
The GIF above shows how products can be added and removed from a cart. The products each have a Consumer which is responsible for displaying how many of that particular product is currently in the cart. They also have a button which accepts the callback onAddToCart
from the Provider.
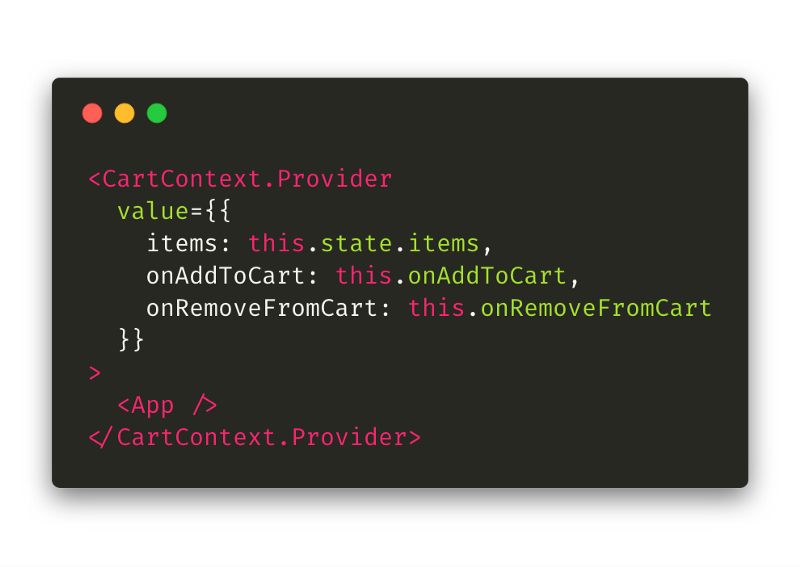
The CartContext
Provider is being rendered by the high level App
component which holds the items in state. This means that the Provider will be updated by a state change and consumers will then update with the new value
object.
The CartContext
has been created in its own file with a default value, so we can import it into the component which will render the Provider as well as the component that Consumes the context:
import React from "react"
export const CartContext = React.createContext({
items: [],
})
Now we can access these values with Consumers like so:
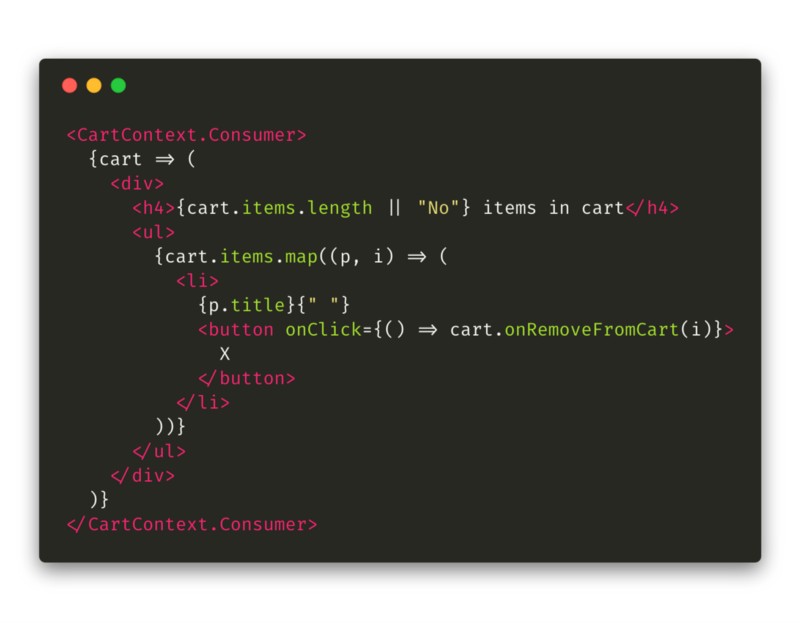
Inside the Consumer, we can now access the value object which is defined in the Provider.
In the sample app, one Consumer is in the header area and is responsible for displaying the number of items in the cart and a list of those product names.
Here is the source for the sample app using the Context API:
contextual - A simple sample app using the React 16 Context API.
Should I use the Context API?
The new Context API can be very useful for pieces of data that are deeply nested within your application and need to be shared. The React documentation recommends it in cases of global state such as the current user, theme or preferred language. These are places that could potentially be in multiple isolated parts of the application and would require a lot of prop-drilling to achieve. This is the perfect scenario.
The sample app is an example of how it could be used for a shopping cart where, as a user, you want to know how many items are in your cart and what they are. Whether or not to use this API depends on the complexity of your application, I would still recommend Redux for most applications — it is a more fully fledged library with a great ecosystem of accompanying middleware that caters to many more challenges. In less complex apps, it seems like the Context API can be very useful and you should give it a go regardless.
For more information on how to use the Context API, the React docs are a great place to start:
Thanks for taking the time to read about the React Context API in v16. Let me know what use cases the Context API can be leveraged for or how you are using it in your own project.
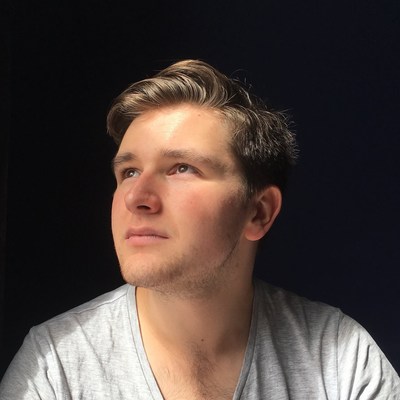
A Fullstack Software Engineer working with React and Django. My main focus is JavaScript specialising in frontend UI with React. I like to explore different frameworks and technologies in my spare time. Learning languages (programming and real life) is a blast.